Built-in functions (Part 1)
Isn't a computer just a glorified calculator?
So far we've talked about numbers and breaking tasks into steps that are simple enough for a computer to understand. Right at our fingertips are steps using functions built into a programming language. This discussion may lead to unkind comparisons between computers and calculators.
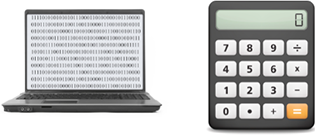
Images: (left) thumb/iStock/Thinkstock; (right) human/iStock/Thinkstock
Like a calculator, a computer processes data, creating new values from old ones.
- A computer is not limited to numbers.
- A computer is not limited to a fixed set of functions.
But, instead of just numbers we can also process movies, sounds, and so on. We are not limited to the buttons on a calculator; we can create our own functions and even our own kinds of data.
Example: determining the cost of a pizza
Suppose we have a computer at our pizza business. Given the diameter of a pizza being ordered and a list of toppings, it determines the cost of the pizza.
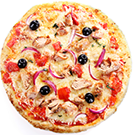
Image: foodandstyle/iStock/Thinkstock
Breaking the task into smaller tasks
We might break this into smaller tasks such as
- obtaining the diameter of the pizza,
- obtaining the list of toppings,
- computing the surface area of the pizza,
- calculating the cost of the dough,
- calculating the cost of one topping,
- adding up the costs, and
- printing the total cost.
Other questions raised
This raises other questions, which we'll consider in the future:
Glimpse of the future
- Can the list of ingredients be of any length?
- How do we look up the cost of a particular ingredient?
Before deciding what functions to use, let's make sure we have the vocabulary necessary to talk about a function.
Describing a function
We can think of a function as a magic box: you put in some data, something mysterious happens inside, and out comes a new value. Here, we put 5 and 3 into the subtraction box and obtain the value 2. We use the terms "input" and "output" to describe the data that goes in and the data that comesout, and say that a function "returns" the output.
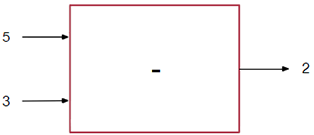
The function takes inputs 5 and 3 and returns the output 2.
You can also think of a function as a miniature factory or small animal, that eats up the inputs and uses them to create the output. From this idea come the terms "consume" and "produce".
The function consumes 5 and 3 and produces 2.
Although in this picture we have two inputs, 5 and 3, in general we could have 0, 1, 2, 3, or more inputs. There is less choice in the number of outputs: we can get back at most one value. Later on we'll learn how to get around this restriction.
In summary, the number of inputs and outputs are as follows:
Inputs and outputs
- Possible numbers of inputs: zero or more.
- Possible numbers of outputs: zero or one.
Some functions make other changes other than returning output, or side effects, such as printing to the screen or changing data.
Tasks using numbers
Since our calculations involve numbers, it is worth asking which functions are provided by the programming language. Not surprisingly, most languages have built-in functions such as arithmetic operations where the multiplication symbol is usually a star instead of the letter x, and the division symbol is a slash.
Mathematical operations: +, -, *, /
Usually these types of operations are written between the data, like in mathematics.
Example: 3 + 4
Common mathematical functions are also built in. Some examples are square roots, powers, and modulo (for positive integers, this is the same as computing the remainder). To use these kinds of functions, usually the name of the function is followed by the inputs in parentheses, separated by commas:
Examples: squareroot(4), power(3, 2), modulo(14, 12)
Strings
It makes sense to represent the size of pizza as a number, but what about a topping? Another common type of data is a string of characters: letters, numbers, symbols, and blank spaces.
Examples: "cat" and "You are number 27 in line!"
Most programming languages have a function that determines the number of characters in the string. As this number is known as the length of the string, in our pseudocode we'll use the name length for this function. For example, to determine the length of the string "OH NO!", we write
length("OH NO!") and it gives 6
In this example there are six characters in total, as we count the blank space and exclamation point. It is the total number of characters, not the number of different characters, so we count both "O"s.
Another common function is known as concatenation, which consists of gluing two strings together to form a new string. Often concatenation is shown using a plus sign. For example, to concatenate the two strings "rain" and "bow", we write
"rain" + "bow" and that resuts in the string "rainbow"
Does that mean that we can use a plus sign on any data? No, most functions work on only specific types of data.
Operator overloading
In operator overloading, the same symbol is used for different operations on different types of data. In this example, the plus sign is used to add numbers or to concatenate strings.
The operator + is used to add two numbers: 3 + 5
The operator + is used to concatenate two strings: "rain" + "bow"
Be aware of when operator overloading is used, and in general make sure you understand the meanings of symbols and functions on different types of data.
Caution
Watch out for operator overloading.
Types of data
Keep in mind that strings and numbers are not the only types of data. In fact, we will learn how to create our own.
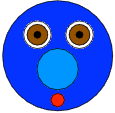
Depending on the language, we may learn a special form to enter the data, so as to distinguish, for example, the number 35 and the string with a 3 and then a 5. There may be another way of displaying the data, and yet another way that the data is stored. In summary, the following may differ:
- How data is entered.
- How data is displayed.
- How data is stored.
How data is entered and how data is displayed are particularly important to learn when you learn a new language.
More functions
- Functions that determine the type of data.
- Functions that form data of a different type.
- Ways to transmit input from and output to a user.
- Ways to request a module of related functions.
Since data types are important, most languages have functions that determine the type of data for you. Sometimes it is possible to create data of one type from data of a different type. In addition, most have functions to allow the user to specify input and ways to print information on the screen. There are sometimes functions available only on request, typically bundled together as a module of functions for a particular type of data.
But what really distinguishes a computer from a calculator is that you can create your own functions to use however you'd like. We'll learn more about breaking tasks into smaller steps, using built-in functions, and, a bit later, creating new functions.