Creating functions (Part 1)
Finding the area of any circle
We have lots of built-in functions - why create new ones? The problem is that not every calculation is already built-in. So we end up writing the same steps again and again. This seems like a wasted opportunity: the computer is fast, so why not have it do as much of the work as possible?
To find out the areas of different circles, we make the same calculation with different values for the radius. Giving the area for each possible radius is a never-ending task:
Calculate π1², Calculate π2², Calculate π3², Calculate π4², Calculate π5², Calculate π6², Calculate π7², Calculate π8², …
Instead, we use a formula to generalize the similar expressions.
f(r) = π r²
Whatever changes, here the radius, can be made into a parameter of a function.
Definition
The changing part in a function is called the parameter.
Apart from the parameter, everything else is the same. This is one example of the idea of abstraction, which comes up a lot incomputer science.
Definition
Abstraction is the process of ignoring the details and finding the common ideas.
Here the details that we are ignoring are the specific values of the radius. The common idea is squaring the radius and multiplying it by pi. Creating a function is one way of using a common idea to solve many problems at once.
The roles played by parameters
In computing the area of the circle, the parameter r played the role of the radius. In this example, there are two parameters, each with a distinct role to play.
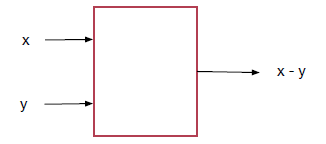
- The role of x is to have y subtracted from it.
- The role of y is to be subtracted from x.
When the function is called on two values, the first is x and the second y. Since y is always subtracted from x, calling the function on 4 and 2 will have a different result from calling the function on 2 and 4. What is important here is not the names but the roles. The “nicknames” x and y specify which input plays which role. The names are chosen just for convenience within the function. It is the order that matters, not the names.
Caution
The order of parameters matters but not the names.
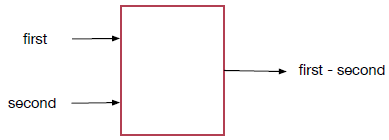
We could have used parameter names "first" and "second" instead of x and y.
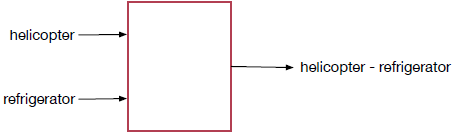
We could have chosen still different parameter names, like "helicopter" and "refrigerator", as long as we subtracted the second value from the first value.
Parts of a function definition
In a mathematical function, we specify the name of the function, the name or names of the parameter or parameters, and the function body, which is how the function is computed. For example, in the function y(x)=x², y is the name of the function, x is the name of the parameter, and the expression on the right hand side of equality, x², is the function body.
How do we choose function and parameter names?
How do we choose function and parameter names? The answer is "just like variables!" That is, for humans, we use meaningful names in a consistent style, and for computers we avoid keywords and other illegalities.
Remember
- Add some meaning to function names.
- Use a consistent naming style.
- Make sure that names are legal.
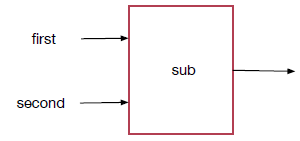
For example, we might use the parameter names first and second and the function name sub.
How do we define a function?
How do we use these to define a function? We can view the function definition as having two parts.
Definition
A function header specifies the names of the function and parameters (and sometimes the types).
Definition
A function body is the code that determines the output.
Most languages have a way to specify the output.
Code examples
Here are a few examples of how various programming languages define functions. Don't worry about the details. In this example, written using C programming language, the parameters and output are all integers:
int sub(int first, int second)
{
return first - second;
}
As in the previous example, return is used to specify the output in this function written in Java:
public static int sub(int first, int second) {
return first - second;
}
In this function written in Python, the data types of the inputs and output are not given:
def sub(first, second):
return first - second
In this function written in JavaScript, the data types of the inputs and output are also not given:
function sub(first, second) {
return first - second;
}
Pseudocode function definition
We'll use a simple pseudocode where define is used to indicate a function definition. A function header consists of the word define followed by the function name and then the parameter names in parentheses, separated by commas.
Here we give the name, sub, followed by the names of the parameters, first and second, in parentheses and separated by a comma.
define sub(first, second)
Remember, it is the order that matters - the order of inputs in the function call must match the order of the parameters in the function header.
Caution
Make sure that the inputs in a function call use the same order as the parameters in the function header.
The function body is indented. We use indentation to show which lines are in the function body. Here there is just one.
define sub(first, second) return first - second
In the future, we will see other situations when indentation is used to group statements.
Glimpse of the future
Indentation is used to group statements in other constructions.
As in the examples, we use return to specify the output.