Creating functions (Part 4)
Flow of control when a function calls a function
To see what happens when we call the function cans, we first need to understand what happens when we call a function, like paint, inside another function, like cans.
Consider a program that needs to call a function, say g, for its task completion. Assume that the function g needs to call another function, say h, for its task completion.
The figures (1)-(6) will be used to depict the process as we describe the execution of the program. The first, second, and third column of circles represent the main program, function g, and function h, respectively. As before, the red circles represent instructions and the bigger red circles represent function calls. The first column of red circles, represeting the main program, consists of four red circles, followed by a bigger red circle to represent the function call to g, and three red circles after the bigger red circle. The second column of red circles, represeting g, consists of three red circles with the middle one being a bigger red circle to represent the function call to h. The third column of cirles, representing h, consists of simply four red circles.
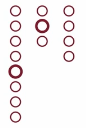
Fig. (1)
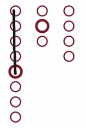
Fig. (2)
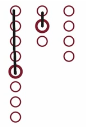
Fig. (3)
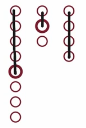
Fig. (4)
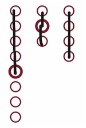
Fig. (5)
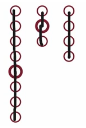
Fig. (6)
Let us now consider how the function f completes its task.
- We execute the code associated with the program until we reach the function call g, then pause while we start executing the function g. In figure (2), this is indicated by the line that begins at top and ends at the bigger red circle, which is the function call to g.
- When the function g encounters a function call to h within the function, we pause there as well. In figure (3), this is indicated by the line that begins at the top of the second column of circles and ends at the bigger red circle, which is the function call to h.
- Next, we execute the instructions in the function h within the function g. In figure (4), this is indicated by the line that begins at the top of the third column of circles and ends at the bottom circle. The bottom circle represents the last set of instructions associated with the function h.
- Then, we complete the instructions in the function g. In figure (5), this is indicated by the line that begins at the bigger red circle in the second column of circles and ends at the bottom circle.
- Finally, we complete the rest of the instructions in the program. In figure (6), this is indicated by the line that begins at the bigger red circle in the first column of circles and ends at the bottom circle.
Calling a function that calls a function
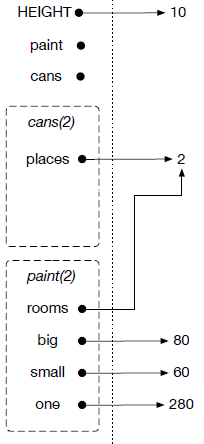
HEIGHT ← 10 define paint(rooms) big ← 8 * HEIGHT small ← 6 * HEIGHT one ← 2 * (big + small) return rooms * one define cans(places) area ← paint(places) coverage ← 5 * 350 return ceiling(area / coverage) number ← cans(2)
In this example, we define two functions, first paint, so we put the name in the address book, and then cans, so we put that name in the address book too. As before, we have only defined the functions, not called them. In the last line of our program, we call the function cans. We create an empty address book, assign the value 2 to the parameter places, and then in order to determine the value of area, we call the function paint. In the temporary address book for paint, the value of rooms is set to 2, since that is the value stored with places. Like in the other example, we assign values to big, small, and one, returning the product of rooms and one, namely 2 and 280, to assign area the value 560.
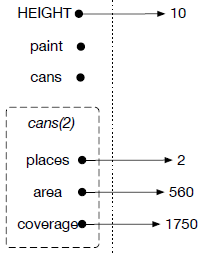
Once area is assigned the value 560, we have completed our function call to paint, so the corresponding temporary address book disappears. This allows us to continue executing the instructions in the function cans, namely assigning a value to coverage.
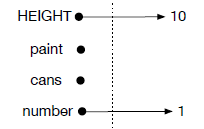
Then number is assigned the ceiling of 560 divided by 1750, which is 1. This completes the our example.