Iteration using while (Part 1)
Repetition in real life
We've talked about using computers to take over repetitive tasks; how do we do that? We first need to be able to detect repetition. Real-life examples don't necessarily have the word “repeat” in them, such as “Eat your fill”. Or if you have instructions on a shampoo bottle, which tell you to repeat lathering and rinsing, but not when to stop, if ever. Or you have driving directions with words like “keep going” to tell you to repeat an action and “until” to indicate when you should stop. Sometimes we will use the term iteration instead of repetition. Each repetition of an action is called an iteration.
Iteration means repetition; one iteration is one repetition.
Finding iteration while eating
How would we express “Eat your fill” as iteration, that is, repetition? The “your fill” part implies that the repetition should stop when you are no longer hungry. We could break this into steps: eat, check for hunger, and then depending on the outcome, either repeat the steps or stop.
Steps involved in “Eat your fill” iteration:
- Eat.
- Ask: Am I hungry?
If “yes”, repeat steps.
If “no”, stop.
Does this idea seem familiar? We can convert the question into a condition. Then, just like in branching, we can check for “true” and “false” instead of “yes” and “no”.
Translation of the “Eat your fill” iteration to statements:
- Eat.
- Condition: I am hungry.
If true, repeat steps.
If false, stop.
Finding iteration while washing
For the shampoo bottle example, you could stop when you run out of shampoo, which might be what the manufacturer would like you to do, or you could stop when your hair is no longer dirty.
Steps involved in “Lather. Rinse. Repeat.” iteration:
- Put on shampoo.
- Lather.
- Rinse.
- Ask the following question and react accordingly: Is my hair dirty?
If the answer is "yes", repeat the steps.
If the answer is "no", stop.
Again, we can convert a question into a statement that is true or false.
Translation of the “Lather. Rinse. Repeat.” iteration to statements:
- Put on shampoo.
- Lather.
- Rinse.
- Condition: My hair is dirty.
If the condition is true, repeat the steps.
If the condition is false, stop.
Visualizing the iteration
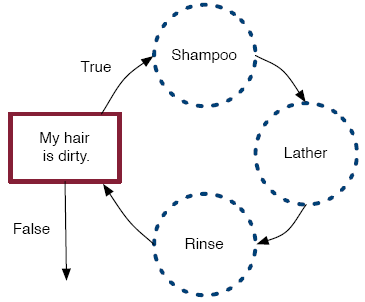
We can think of the steps of putting on shampoo, lathering, and rinsing as occurring in that order, with the condition being checked both before the three steps start and after the three steps finish. The two outcomes of checking the condition are executing the steps again if it is true and stopping if it is false.
Finding iteration while driving
The driving directions tell you explicitly what to do after the repetitive part. Since we prefer to turn the car onto a street rather in the middle of block, at the end of the block, we will look for the ocean. The most natural question here results in repetition for a no answer instead of a yes answer.
Steps involved in “Keep going until you see the ocean, then turn left.” iteration:
- Drive a block.
- Look for the ocean.
- Ask the following question and react accordingly:
Can I see the ocean?
If the answer is “yes”, repeat the steps .
If the answer is “no”, turn left.
To keep the same pattern as the other examples, that is, to have repetition occur when the condition is true, we will choose a condition that is true when the answer is no and false when the answer is yes.
- Drive a block.
- Look for the ocean.
- Condition: I cannot see the ocean.
If the condition is true, repeat the steps.
If the condition is false, turn left.
Visualizing the iteration
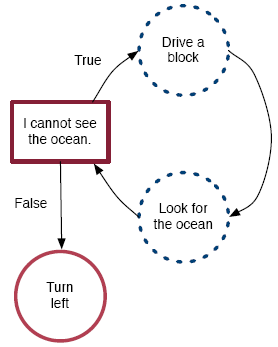
As before, we put the steps in order and put the condition both before and after the steps. If the condition is true, the steps are repeated, and if it is false, the next step, namely turning left, is executed.
Discussing iteration
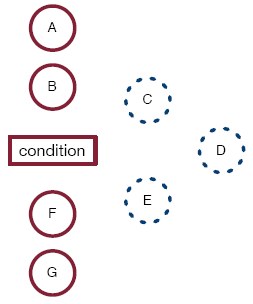
Using a generic visualization as an example, how might we describe what is going on? The first few steps that precedes the condition can be represented by red circles. Next comes the condition, represented by a red rectangle, and the loop body, represented by dashed blue circles. The loop consists of a condition and a loop body or body of the loop. Finally. the steps that come after the loop can be represented by red circles.
For example, consider an iteration that has two steps before the condition, say A and B; three steps in the loop body, say C, D, and E; and two steps after the condition, say F and G. The visual representation consists of A and B represented by two red circles; the condition represented by a red rectangle; C, D, and E represented by three dashed blue circles; and F and G represented by two red circles. We describe the repetition as a while loop, since the dashed blue circles form a series of steps that form a loop and the loop is repeated while a condition is true. In our driving example, turning left was a step that came after the loop. None of the examples had steps that came before the loop. The loop itself consists of the condition and the blue dashed circles, which are known as the loop body, or body of the loop. We'll look in more detail about how to use while loops in writing programs.