Iteration using while (Part 2)
Translating from pictures to pseudocode
Consider again the iteration that has two steps before the condition, say A and B; three steps in the loop body, say C, D, and E; and two steps after the condition, say F and G. The visual representation consists of A and B represented by two red circles; the condition represented by a red rectangle; C, D, and E represented by three dashed blue circles; and F and G represented by two red circles.
How might we translate our visualizations to pseudocode? We use the keyword while to introduce the condition. Indentation is used to show that steps C, D, and E are blue dashed steps in the body of the loop. These four lines, while with the condition, C, D, and E, form the while loop. Steps before the condition come before the loop and steps after the condition come after the loop.
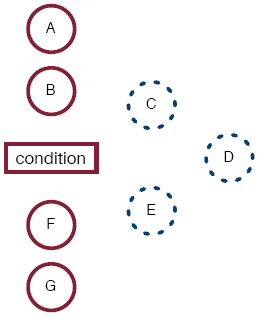
A B while condition C D E F G
If the condition is never true, the order of execution of steps is A and B, followed by a check of the condition, and since the condition is never true, the next steps are F and G:
The order of execution if the condition is true 0 times is A, B, condition, F, and G.
If instead the condition is true exactly once, steps A and B are executed, the condition is found to be true, so the steps in the loop are executed, and then the condition is checked again. This time it is false, so the final steps are F and G:
The order of execution if the condition is true 1 times is A, B, condition, C, D, E, condition, F, and G.
We will look at one more case, in which the condition is true exactly twice. Again we start with A and B and then reach the condition. The condition is true, so we go around the loop to execute C, D, and E, and end up at the condition again. The condition is true a second time, so we go around the loop again, and again end up at the condition. This time the condition is false, so we end with F and G.
The order of execution if the condition is true 2 times is A, B, condition, C, D, E, condition, C, D, E, condition, F, and G.
In general, if the condition is true n times, it is checked n+1 times, and in all but the last time, the loop is entered.
While loop
Ignoring the steps before and after the loop, we can write a loop in the following form, where the body of the loop can be any number of steps:
while condition body of the loop
In the first example, the condition was "I am hungry" and there was a single step in the body of the loop, namely "Eat":
while I am hungry Eat
In the hair washing example, we had three steps in the body of the loop:
while my hair is dirty Put on shampoo Lather Rinse
Finally, in the example of "driving until the ocean is seen," we have two steps in the body of the loop, and one step outside the loop after the execution of the loop is over:
while I cannot see the ocean Drive a block Look for the ocean Turn left
Setting conditions
The condition dictates the number of iterations, that is, the number of times through the loop. If the condition is always false, then the steps in the body of the loop are never executed.
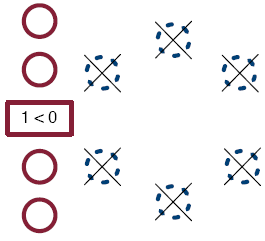
while 1 < 0 Eat
On the other hand, if the condition is always true, the iterations continue forever in what is called an infinite loop:
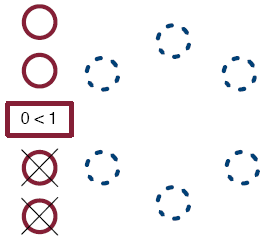
while 0 < 1 Eat
Infinite loops
An infinite loop repeats forever.
In particular, it means that no steps after the loop are ever executed.
Making a condition false using user input
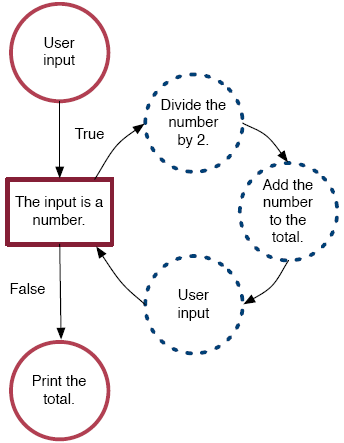
How can we make it possible for the value of a condition to change? That is, in some cases it can initially be true, so that the loop is executed at least once, and then eventually become false, so that it does not go on forever. One way is to have the value of the condition depend on user input. In this example, the condition is false when the input is not a number.
Making a condition false by changing a variable
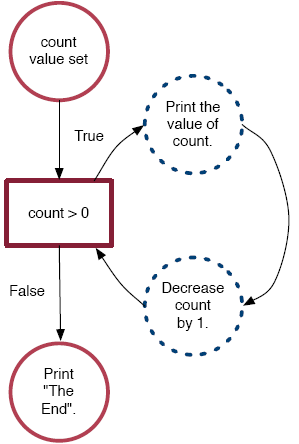
Another way to make the condition change is by allowing a step or steps in the body of the loop to change a variable that appears in the condition. Here, the variable counter is decremented each time through the loop, with the condition being false when the value is no longer positive.
Changing a variable in more detail
It is crucial that every time the condition is checked the variable has a value. This means ensuring it is given a value before the loop is entered.
Caution
The variable must have a value before the condition is checked.
We can trace through this loop for the case in which the value of count is set to 4 before the condition is checked. The first time the condition is checked, the condition is true, so the loop is entered and the value is updated to 3. The second time the condition is checked, the condition is still true, so the loop is entered again and the value is updated to 2. On the third checking of the condition, again the condition is true, the loop is entered, and the value is updated, this time to 1. The fourth time the condition is still true, so the loop is entered yet again and the value updated. At the fifth time the condition is encountered, the condition is no longer true, so the loop is not entered. Notice that the number of times that the loop is entered is one less than the number of times the condition is checked. This is summarized in the table below.
Loop attempt number | Count before body | Does it enter the loop? | Counter after body |
---|---|---|---|
1 | 4 | Yes | 3 |
2 | 3 | Yes | 2 |
3 | 2 | Yes | 1 |
4 | 1 | Yes | 0 |
5 | 0 | No |