Introduction
Python from scratch
Welcome!
Whether you are a beginner interested in learning to program or someone needing review of a particular programming concept, you've come to the right place. All the materials are designed for you to use at your own pace. You might want to watch some videos more than once, or to pause in the middle to try out an exercise on your own. We've also provided extra features to facilitate exploring and experimenting on your own.
Site overview
You might be wondering which programming language makes most sense to learn. The good news is that it doesn't really matter that much. The basics are all pretty much the same. To make it easier for you to distinguish what is true of programming in general and what is true about a particular language, I have separated the instructional material into language-independent and Python-specific videos.
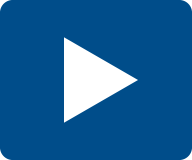
Language-independent
video icon
The language-independent videos, which do not have pythons in their icons, give an overview of the concepts being taught, without assuming what programming language you are learning.
We've grouped them together for easy access by those who might wish to use these materials for review or for studying languages other than Python. They are accessible through the Open CS Home page under the title Language independant lessons.
Screenshot of Open CS home page, with Language independant lessons highlighted.
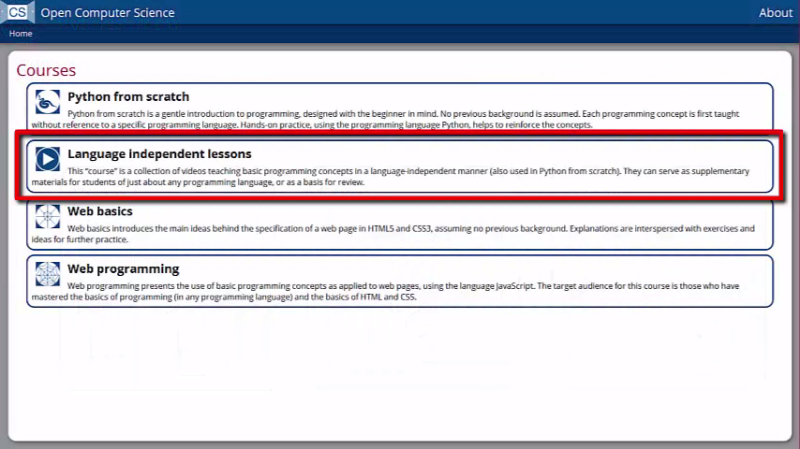
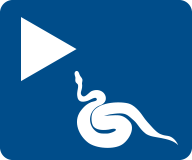
Python video icon
The Python-specific videos use the programming language Python to further explore the concepts introduced in the language-independent videos. These include demonstrations of examples, giving you the choice of watching, programming along with the video, or revisiting the material on your own afterwards.
The materials for Python from scratch are divided into modules. Each module is made up of a sequence of steps, consisting of two types of videos to explain the concepts and two types of exercises to reinforce the concepts. Although these can be completed in any order, you are encouraged to try the exercises in the order given. You can stay on an exercise as long as you like.
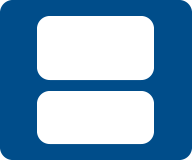
Exploration icon
This icon represents a Python panel, which is used in hands-on exercises in writing programs. In some of the exercises there are optional hints for you to use if you wish; these can be viewed by clicked the provided Show Hint button in the Instructions panel.
Screenshot of an exploration. The Show Hint button is located at the end of the Instructions panel.
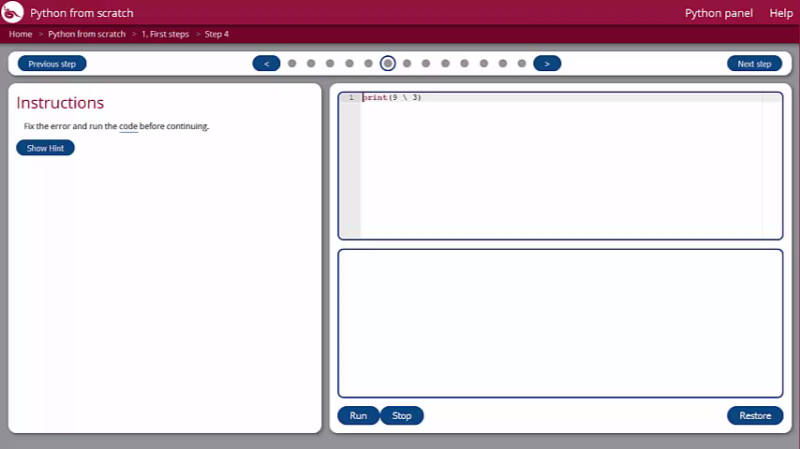
When you submit your solution, it is automatically checked. If it is correct, a green checkmark appears. If it isn't quite right, we try to provide some indication of what you might have overlooked.
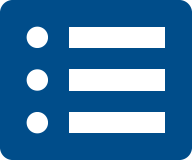
Quickclick icon
You can exercise your mind in a slightly different way using quickclicks, which give you practice in the form of multiple choice and short answer questions with feedback. These exercises are designed to increase, not test, your knowledge. Don't expect to get the correct answers right away. We provide you with hints for wrong answers, and feedback on correct ones, both designed to help you learn.
Navigation
Although we recommend covering all materials in order, you may wish to navigate back through earlier material for review. We've structured navigation in the course so that you can first choose a course, then a module, and then a step.
Screenshot of the Open CS home page. It contains a list of courses to choose from.
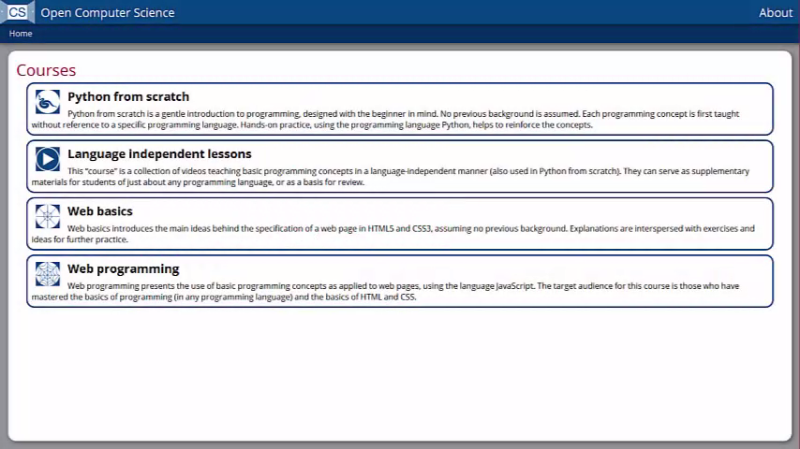
Screenshot of the Python from scratch page. It contains a list of modules to choose from.
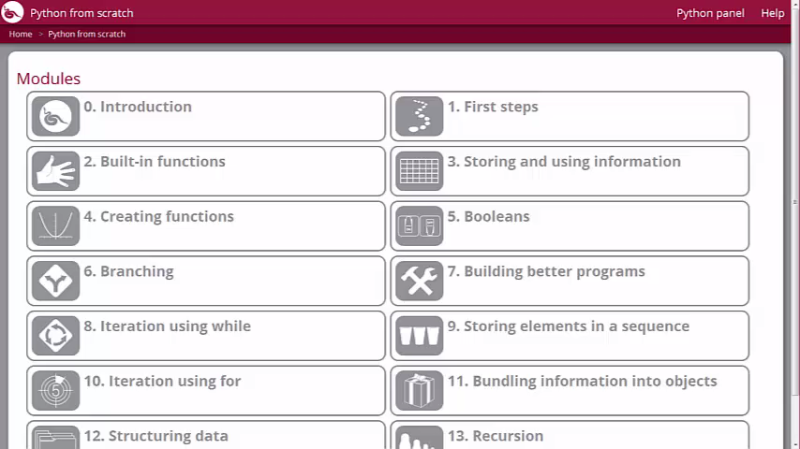
Screenshot of a module page in Python from scratch. It contains a list of steps to choose from.
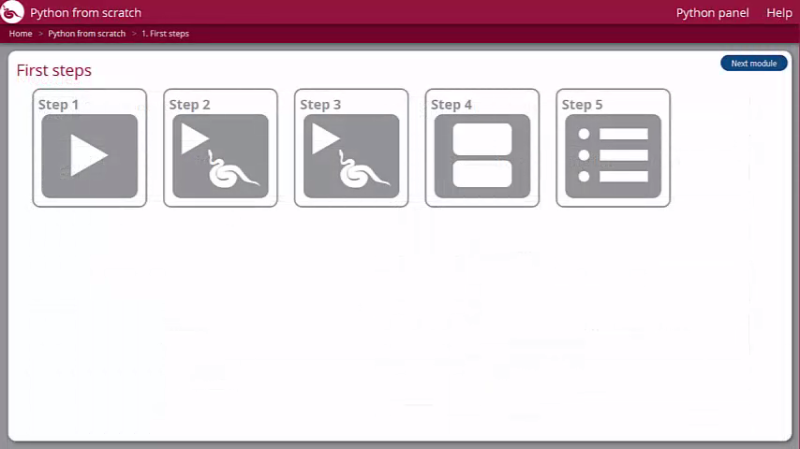
Screenshot of a step page. Breadcrumbs which sit in the navigation bar are highlighted.
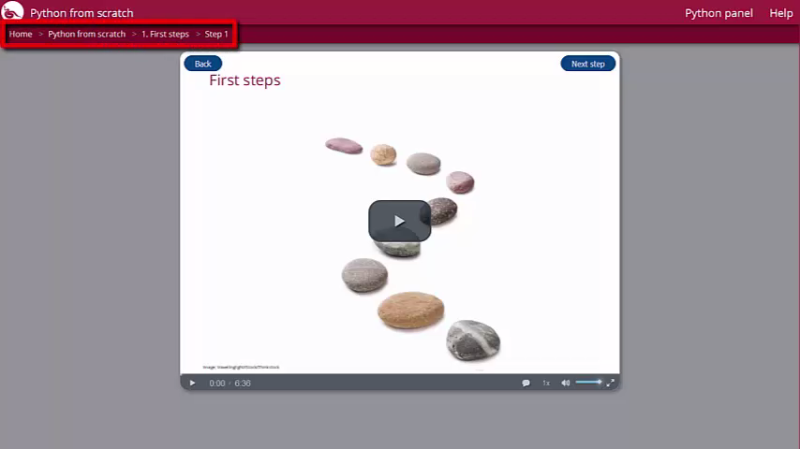
The breadcrumbs in the navigation bar give a record of where you are and an easy way to backtrack.
Screenshot of a step page. The Next step button in the upper-right corner is highlighted.
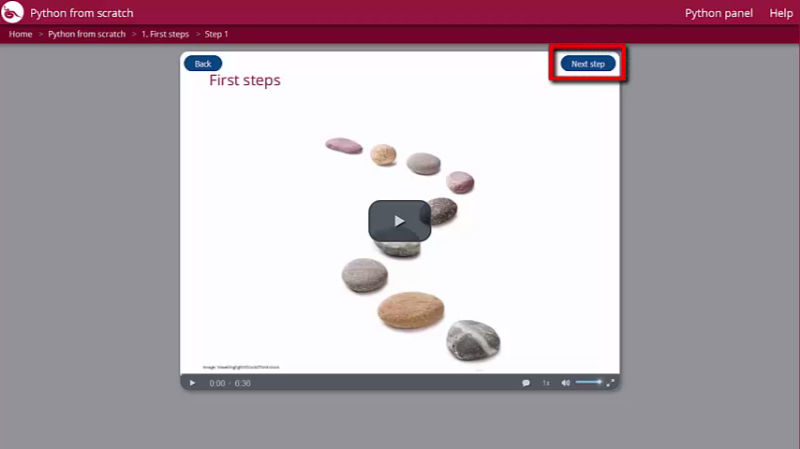
We've also provided you with a button labelled Next step that will take you to the next step in the same module.
In the explorations and quickclicks, you can navigate to earlier or later exercises by using the buttons marked with arrows, or by clicking on the progress bar, which is color-coded to let you know which exercises have been completed and which exercises have been attempted but not yet completed.
Using a Python Panel
The course makes extensive use of Python panels, which appear in the video demonstrations as well as in the exercises. There is also a Python panel accessible from the menu, which you can use at any time, whether to program along with me, to revisit code seen in lectures, or to try your hand at programming anything you like.
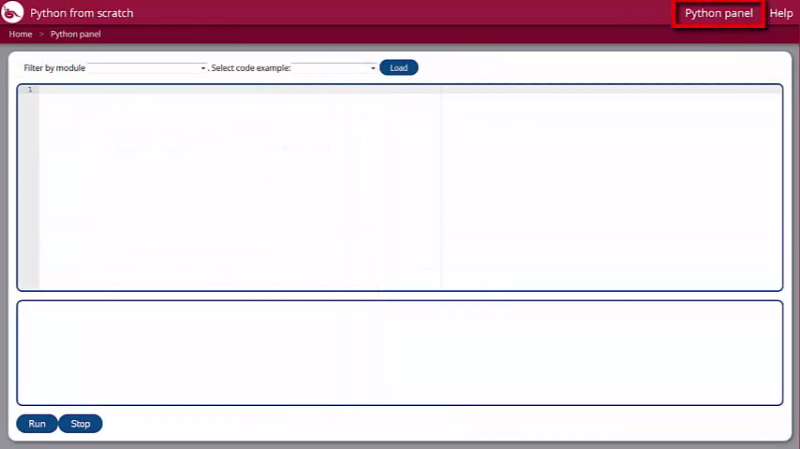
Each python panel has an upper box, where a program is entered, and a lower box, used for interaction. You can access a Python panel at any time from the menu. To load a exercise shown in the video, you can use the module number as a filter.
There are few handy features to help make it easier for you to enter a program. Syntax highlighting is used to show the uses of various special words in Python. For example, when I type the word print
into the Python panel, the colour of the word changes to burgundy. When a close parenthesis is entered, a little box appears around the matching open parenthesis. This is a good opportunity for you to check to make sure that it is matching up the way that you think it should and add or delete parentheses as needed to fix any errors that you discover.
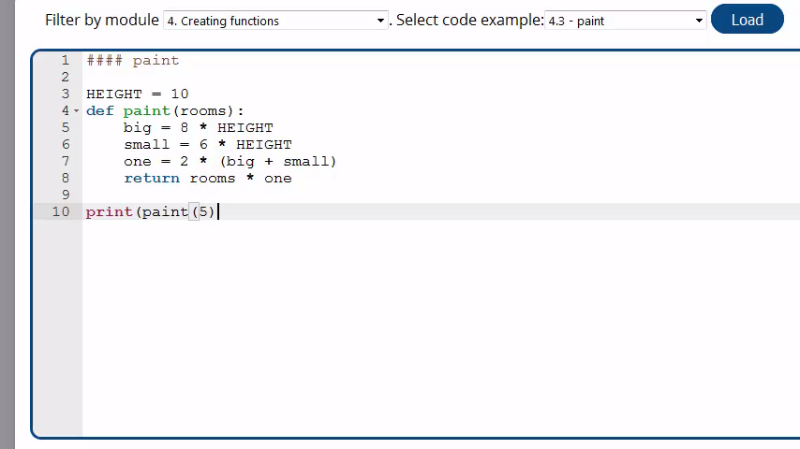
There are also light lines to show different levels of indentation.
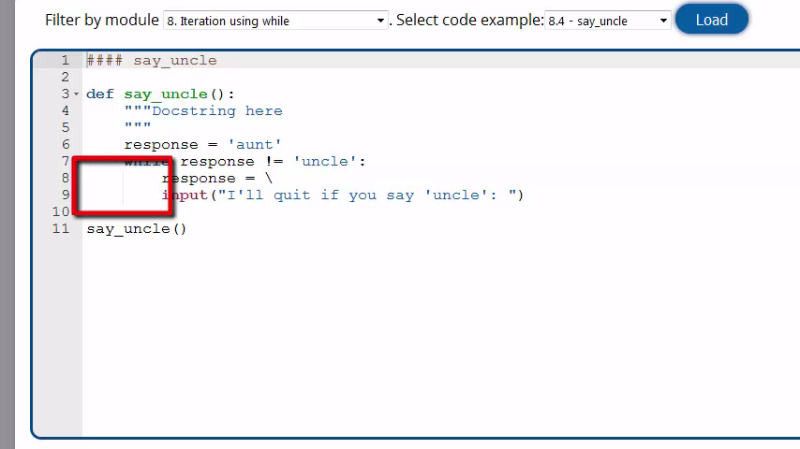
Each Python panel has a Run button, which you should click when you're ready to run the program you create. The Stop button comes in handy when you've accidentally asked the computer to execute tasks forever. If you aren't getting a response for a long time, you might want to make use of that button. If you program works correctly, or even if it doesn't, you will see the result in the lower box.
#### chocolate sales
BAR_COST = 2 # cost of a bar of chocolate
def is_yes(word):
return word[0] == "y" or word[0] == "Y"
## Ask user for number of bars
bars = int(input("Enter number of bars: "))
## Compute cost of chocolate
cost = bars * BAR_COST
## Ask user if donation desired
donate = input("Donate $10? ")
if is_yes(donate):
cost = cost + 10
print(cost)
After typing this program and clicking the run button, the text Enter number of bars: appears in the interactions panel, and an input box appears. When 3
is typed, the text Donate $10? appears in the interactions panel, and an input box appears. When y
is typed, 16 is shown. The resulting interactions panel looks as follows:
Enter number of bars: Donate $10? 18
def factorial(num):
for i in range(num):
prod = prod * (i + 1)
return prod
def test_factorial():
assert factorial(0) == 1
assert ordinal(1) == 1
assert ordinal(2) == 2
assert ordinal(5) == 120
test_factorial()
After typing this program and clicking the run button, the following error appears in the interactions panel.
Traceback (most recent call last): File "<string>" line 12, in <module> File "<string>" line 7, in test_factorial File "<string>" line 4, in factorial UnboundLocalError: local variable 'prod' referenced before assignment
If you don't understand it, go to the help page, to find a list of explanations of error messages.
For some of the exercises, the code box has in it a program that you can modify. If you decide that you don't like the changes you've made, you can use the Restore button to have the original program reloaded. But be careful; clicking this button will cause you to lose any of the changes you have made.
Other features
Whenever you need more information, go to the help page, which will direct you to resources in this course and beyond.
Screenshot of the Help page. The Help button in the navigation bar is highlighted.
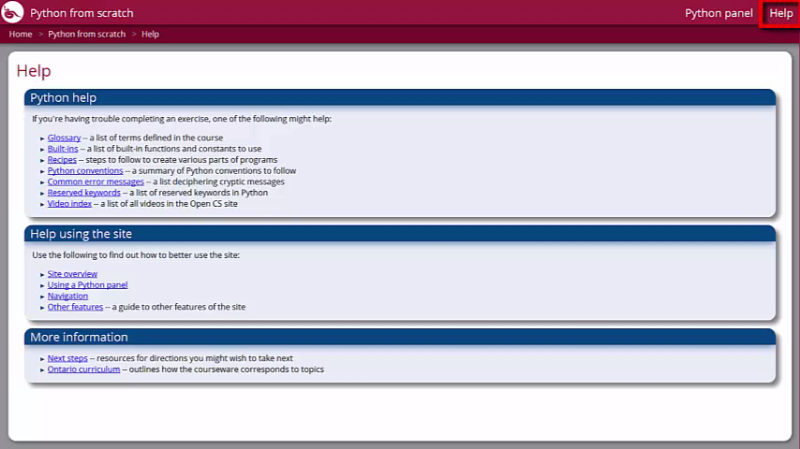
For a reminder of a term used in an exercise, hover over it to see the definition.
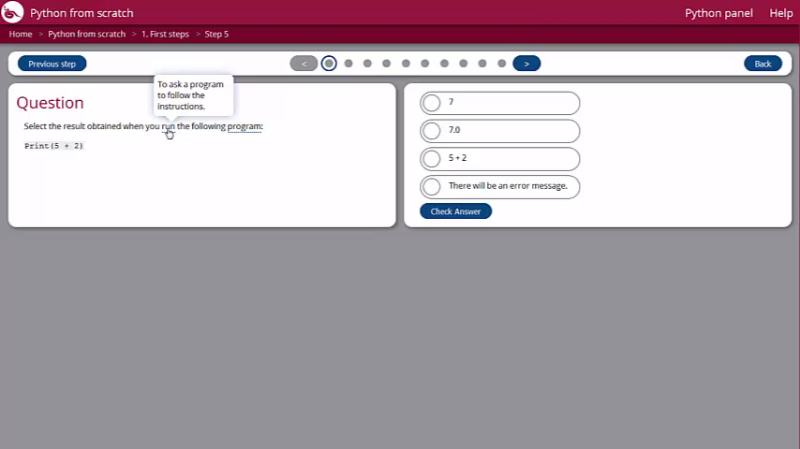
A list of glossary terms is also available.
Here you can also find a list of all the built-in functions taught in the course. You can access them in order of their names, their descriptions, and the order in which they appear in the course.
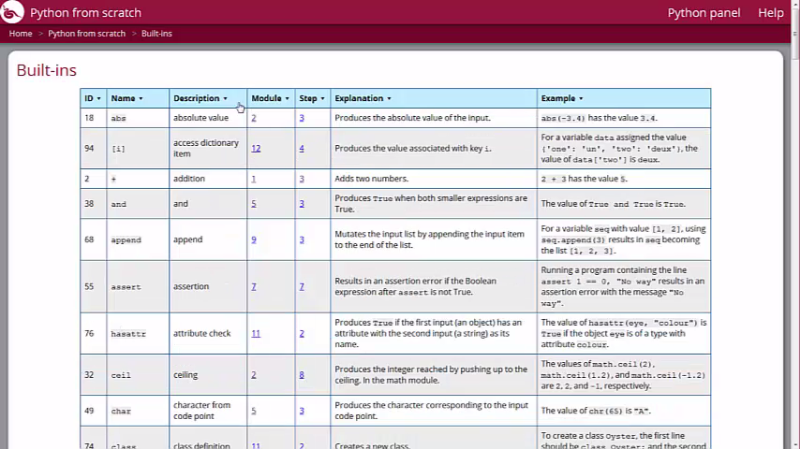
Various ideas that are summarized as recipes in the lectures are collected on the Recipes page.
Other reference materials provided for your convenience include conventions used in writing Python, a list of common error messages, and keywords to avoid. We also have links to external resources as directions to explore should you wish to learn more after completing the materials we have here.