First steps in Python (Part 1)
Why Python?
So why is the course being taught in Python?
Not reasons
- Named after a comedy group.
- Snakes.
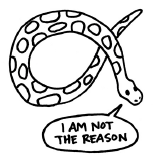
It is not because of the way the name was chosen, and it is not because of all the opportunities to use images of snakes.
Reasons
- Simple and easy to learn.
- Lots of materials available for further study.
- Widely used in many real-world settings.
It is because it is a great language for beginners. You can start in on interesting ideas instead of getting tangled up in syntax. Since it is so popular, there are lots of materials available if you want to learn even more about it. That in turn will allow you to apply your knowledge widely. So let's get started!
Different types of numbers
Definition
An integer is a number that can be written without a fractional part. In Python, the exact value is stored.
Python distinguishes between numbers that are stored exactly and ones that are stored as approximations. An integer is a number without a fractional part, i.e., there is no decimal point with digits following it. Python uses exact values of integers. An integer can be positive, zero, or negative. For example, 5
, 0
, and -402
. Creating an integer in Python is easy: just don't use a decimal point.
Definition
A floating point number is a number stored in a fixed amount of space. In Python, the value stored might be approximate.
A floating point number is a number stored in a fixed amount of space. If the number of digits in a number cannot fit into the fixed space, then the value stored will be an approximation. Again, we can have positive, zero, or negative floating point numbers. For example, 11111111111.
, 0.0
, and -.222222222222222
. As you can see in the first two examples, there doesn't have to be fractional part for a number to be a floating point number. It is the use of a decimal point that distinguishes a Python floating point number from a Python integer.
Entering integers
All demonstrations and explorations will make use of a Python panel.
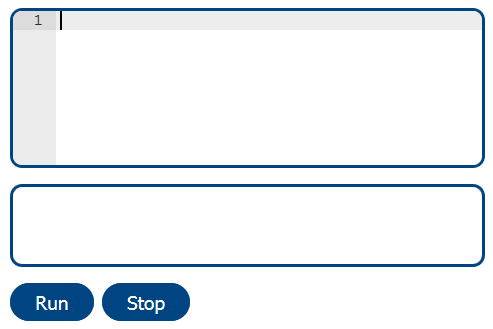
There is also a tab on the main menu to take you to a Python panel that you can use at any time. We'll call the top box a “code box” since this is where we'll enter code, i.e., instructions to the computer. The bottom box, or “run box”, shows results from running the program. When a computer runs a program, it follows (or "executes") the steps in the program. To run a program, that is to run the program in the “code box”, click on the run button. For example, type the number 45 in the “code box” and click the run button. Why isn't there a result in the “run box”? I typed a number, but didn't tell the computer what to do with it. To print a number to the screen, I write the word “print” and put 45 in parentheses to show that it is 45 that we'd like to print:
Print(45)
The figure below shows how this statement looks when typed in the “code box”.
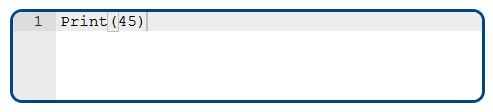
The strange-looking box around the open parenthesis is showing that it matches the close parenthesis.
You can use this features to help make sure you are typing accurately. The following error is generated when the code Print(45)
is run.
Traceback (most recent call last): File "<string>", line 1, in <module> NameError: name 'Print' is not defined
What's wrong now? This is an error message. Sometimes the details give useful information, sometimes not. The first lines of the message indicate where the error took place; it is often useful to look at the last line, which indicates what went wrong. The error message tells us that Print
is not defined. How could that be? Remember that the computer needs very precise instructions. It understands the statement print
when it is written in lower-case letters. Capitalizing the first p is enough to confuse it. Let's try typing the word “print” with all letters in lower case.
The figure below shows how this statement looks when typed in the “code box”.
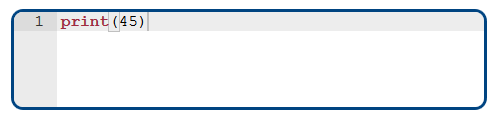
Notice how the colour of the the text changed from black to burgundy when the word Print
was changed to print
. This is called syntax highlighting: different colours and fonts are used to make clear the roles of various parts of the code. The colours make no difference to the computer; they are used to make the code clearer to humans. When print(45)
is typed, we got what we want: the computer displayed exactly what we entered, 45.
Let's try again, this time with a negative integer. The statement print(-5)
gives the output -5.
What about a larger number this time? The statement print(3,123)
gives the output 3 123. Something strange happened - we'll learn later how the computer interpreted what we wrote. For now, remember to leave commas out of your numbers. So let's try again without the comma. The statement print(3123)
gives the output 3123.
What if we added some extra blank spaces? The statement print(3123)
gives the output 3123. This time the computer did not display exactly what we entered, since it ignored the blank spaces.
What if we type the statement print( 31 23 )
? This gives following errors.
Traceback (most recent call last): File "<string>", line 1 Print( 31 23 ) ^ SyntaxError: invalid syntax
This time the computer did not ignore the blank spaces. The blanks separated the number into two numbers. The error message tells us that the syntax for print
does not allow us to provide two values in this way.
Entering integers: what we learned
Here's a summary of what we learned about programs that use integers.
Definition
To run a program is to ask the computer to follow (or execute) the instructions.
Syntax
- Print a value using print in lower case, with the value in parentheses.
- Use - for a negative number.
- No commas.
- No spaces between digits.
Printing only works when we use lower case, with the value in parentheses. We can make negative numbers by putting a dash in front as a minus sign. We should avoid commas when writing large numbers or numbers of any other size, for that matter, and we will get an error message if we leave spaces between digits.
Entering floating point numbers
Let's try some different ways of entering a decimal number. Entering the following
print(2.000000000000001)
print(2.00000000000000000000001)
gives the output
2.000000000000001 2.0
In Python, as soon as you use a decimal point, you are entering a floating point number. This means that what is stored might be an approximation. The amount of space being used to store numbers is big enough to keep the last one in the first number, but not in the second number. You may have noticed that the current line is highlighted in gray:
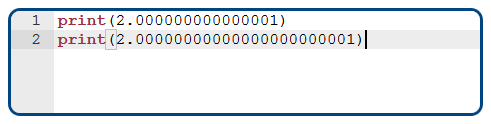
Sometimes I'll use blue highlighting to draw your attention to particular lines:
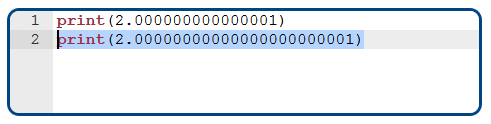
The following code
print(0.8)
print(000.8)
print(000.800000)
print(-0.8)
gives the output
0.8 0.8 0.8 -0.8
In this set of examples, notice how the number of leading or trailing zeroes does not make a difference, and how a negative number is entered by starting with a minus sign.
What if instead we wanted to enter the number as a fraction? The code print(4/5)
gives the output 0.8. We get the right answer, since the slash is interpreted as division.
Entering floating point numbers: what we learned
Syntax
- Use a decimal point for a floating point number.
- Approximations may be used.
- Use - for a negative number.
- Using / for fractions is the same as dividing.
We have seen that using a decimal point signals to the computer that what is entered is a floating point number, and that you are allowing it to be stored as an approximation. Just like for integers, we create a negative number with a leading dash. We've seen that using a slash to write a fraction is really nothing more than dividing the numbers, which is then displayed to us in decimal notation.