Built-in functions in Python (Part 4)
More string functions
There are many more string functions. We will introduce them gradually as we use them. Here are a few that will come in handy:
Function | Result | Python |
---|---|---|
upper case | UPDOWN | 'UPdown'.upper() |
lower case | updown | 'UPdown'.lower() |
Upper creates a new string in which all lower case letters are replaced by upper case letters, leaving all other characters unchanged. Lower creates a new string where all upper case letters are changed into lower case letter and all other characters unchanged.
Caution
- Only lower case letters can be changed into upper case letters.
- Only upper case letters can be changed into lower case letters.
- No other changes occur.
Caution
These functions use dot notation.
Notice that these functions use dot notation, so that the function names follow the input.
Using string functions
Here are a few examples using the slice function. The following code
print('string'[2:])
print('string'[:2])
print('string'[2:4])
print('string'[2:2])
gives the output
ring st ri
Notice what happens when the two slice positions are the same - we have thrown away everything to the left and everything to the right, so nothing is left.
Now consider the following code
print("Five to 10?".upper())
print("Five to 10?".lower())
which gives the output
FIVE TO 10? five to 10?
These uses of upper
and lower
demonstrate how numbers and punctuation marks are unchanged. Notice that upper
does not replace upper case letters and lower
does not replace lower case letters.
Example: a simple code
Now that we know how to handle strings in Python, let's go back to our example. We wish to exchange the first and middle characters of a string. We create our coded string by concatenating the four pieces in this order:
- middle character,
- substring from second to one before the middle,
- first character, and
- substring from one after the middle to the last.
Caution
The string cannot be the empty string.
But we won't be able to make slices of an empty string, so we add the extra condition that the string is nonempty. We also need to know what the floor function is in Python.
Making integers
There are three functions of interest: ceiling, floor, and truncation. We've already seen how ceiling and floor behave: ceiling pushes a number up and floor pushes a number down. Truncation cuts off everything after the decimal point. For example, the following code
import math
print(math.ceil(-1.1), math.ceil(-1.9))
print(math.floor(-1.1), math.floor(-1.9))
print(math.trunc(-1.1), math.trunc(-1.9))
gives the output
-1 -1 -2 -2 -1 -1
For all numbers between -2 and -1, ceiling pushes them up to -1, floor pushes them down to -2, and truncate cuts everything off except the -1, which means that the number gets bigger. Let's consider another set of numbers. The following code
import math
print(math.ceil(1.1), math.ceil(1.9))
print(math.floor(1.1), math.floor(1.9))
print(math.trunc(1.1), math.trunc(1.9))
gives the output
2 2 1 1 1 1
For numbers between 1 and 2, notice that now truncate makes the number smaller.
Function | Pseudocode | Python |
---|---|---|
ceiling | ceiling(4.2) | math.ceil(4.2) |
floor | floor(4.2) | math.floor(4.2) |
truncate | math.trunc(4.2) |
Notice that again we see a function in pseudocode that has a shorter name in Python.
Optional information
The int
function converts floating point numbers to integers using truncation.
If you thought the behaviour of truncation seemed familiar, you were right: it is what the int
function does when forming integers from floating point numbers.
Finding the middle character
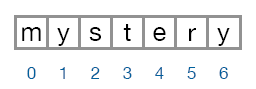
How do we express the new string in Python? For example, consider the string “mystery”.
First, we need to find the middle character. What happens if we find the length of string and divide it by two?
len("mystery") / 2
Since 7 divided by 2 is not an integer, we use floor to transform it to the integer 3:
math.floor(len("mystery") / 2)
In fact, dividing by 2 does not result in an integer even for a string of even length, since division always returns a floating point number. We can now use the index to extract the middle character:
"mystery"[math.floor(len("mystery") / 2)]
Forming the new string
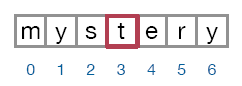
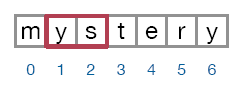
Now that we know the index of the middle character, math.floor(len("mystery") / 2)
, we can also extract the substring that starts at position 1 and goes to one before the middle, because we can use the middle index as a slicing point:
"mystery"[1:math.floor(len("mystery") / 2)]
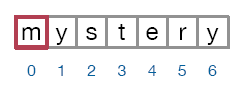
Finding the first character is refreshingly simple:
"mystery"[0]
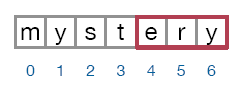
We use slice again for the second substring, which is a suffix:
"mystery"[math.floor(len("mystery") / 2) + 1:]
We cut away everything to the left of one after the middle.
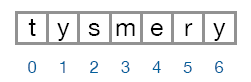
Finally, we put all the pieces together:
print("mystery"[math.floor(len("mystery") / 2)] + \
"mystery"[1:math.floor(len("mystery") / 2)] + \
"mystery"[0] + \
"mystery"[math.floor(len("mystery") / 2) + 1:])
Notice that to continue to another line, we use a backslash \
.
Tricky parts about using strings and functions
Remember ...
- Trailing blanks may be hard to see.
- Be careful of indices in the slice operation.
- Syntax may differ.
- The empty string is a special case.
In using strings and functions, watch out for these stumbling blocks: blanks at the end of a string may not be visible since no quotation marks are used for display. The slice
operation refers to slicing points, not the positions that are included. Keep track of which functions use dot notation, like upper
and lower
. Not every function works on the empty string, so watch out for that case, if it can occur.
Remember ...
- Check types of inputs.
- Check orders of inputs.
When using functions for any type of input, make sure that the input or inputs are of the right type or types, and make sure that the order of inputs is correct.