Built-in functions (Part 2)
Applying function to expressions
Now that we've talked a bit about how to break a task into smaller steps, and what kinds of built-in functions to expect, we can address the question of how to put the smaller steps together to accomplish our task.
In mathematics, we know that the plus sign doesn't need to go between two numbers, but can instead be put between two expressions, such as in 3² + 4².
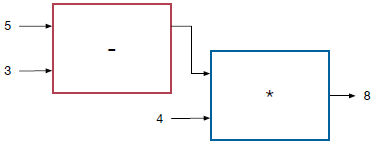
Using functions on expressions
In this way we can think of functions as building blocks that can be put together in many different ways. That is, we can connect up inputs and outputs of our boxes.
Function calls
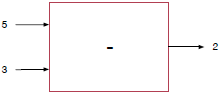
To be able to describe this clearly, we need a bit more terminology. Here is the function we saw before.
The instruction to use the subtraction function on 5 and 3 is called a function call. We say that we call the subtraction function on the inputs 5 and 3.
Other terms
There are other equivalent terms, such as calling the instruction
- a function application, in which we apply the function to 5 and 3, and
- a function invocation, in which we invoke the function on 5 and 3.
Don't worry about learning all these terms; we will not use the equivalent terms in this course. We will only use the terms function call and call in this course.
Common syntax for function calls
To be precise in describing how we use our building blocks, let's look a little more carefully at how we represent function calls.
We've already seen that for operations, we usually write the symbol between the inputs. The first example has two inputs and the second only one.
Examples:
- Operation between inputs: 3 + 5 outputs 7.
- Operation before input: - 5 outputs -5.
We've also see other functions where the name is followed by the inputs in parentheses, separated by commas.
Examples:
- The function squareroot(4) outputs 2.
- The function modulo(14, 12) outputs 2.
There is another syntax that you'll sometimes see, known as dot notation. Here the input comes first, followed by a period (or dot), and then followed by the name of the function. This notation can also be used for a function with more than one input. The first input comes before the period and the rest of the inputs in parentheses after the name of the function.
Examples:
- The operation "bow".upper() outputs "BOW".
Here, the function "upper" creates a string which is like the input string but every lower-case letter is replaced by an upper-case letter. The input string appears first, then a dot, and then the name of the function. - The operation "bow".replace("w", "y") outputs "boy".
In this example, a new string is created in which any character matching the second input, that is w, is replaced by the third input, that is "y".
Operation composition
Using our box notation, we can think of the output of one box being the input to another box. To combine two operations, we use an expression as an input.
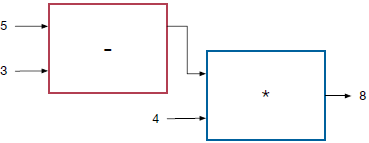
Here, we put the use of subtraction in parentheses to show that the entire expression is the first input to multiplication: (5 - 3) * 4.
Function composition
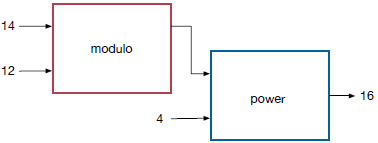
The same idea holds for combining functions. We can use the result of a function call as the input to another function.
The output of modulo is the first input to power: power(modulo(14, 12), 4)
As before, the inputs to a function appear in parentheses to the right the name of the function; this expression represents the function call to modulo. We then put the function call to modulo inside the function call to power, since the output to modulo is an input to power
Caution
The last function called appears farthest left in the expression.
In this syntax, we put the name of the function to the left of its inputs. We first call modulo, and then use it as an input to power, with the result that power is to the left of modulo.
Composition using dot notation
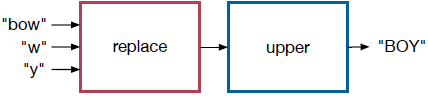
In this example, the output of replace is used as the input to upper.
The output of replace is the input to upper: "bow".replace("w", "y").upper().
Since the input should appear before the period in dot notation, this is expressed as the output for replace followed by a period followed by the function upper.
Caution
The last function called appears farthest right in the expression.
In contrast to what we saw with the previous syntax, here a function is placed to the right of its inputs. We first call replace and then put upper to the right, since the output to replace is the input to upper. Here replace and upper appear left to right in the order in which they are called.
More composition
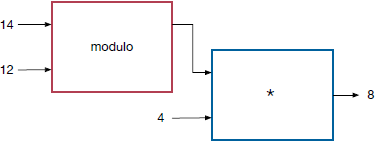
We can mix together different types of syntax in our composition. Here we use both the modulo function and multiplication.
The output of modulo is the first input to the multiplication: modulo(14, 12) * 4.
Functions as building blocks
I hope that the main idea was not lost in all this notation. We can combine functions in different ways. That is, we can use functions inside of other functions to accomplish all sorts of tasks, combining them in different ways for different purposes. Even though we're not yet ready to start our pizza business, we'll get there, step by step.