Built-in functions in Python (Part 1)
Example: surface area of a pizza
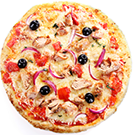
Image: foodandstyle/iStock/Thinkstock
Let's start with one of the subtasks that we identified, namely figuring out the surface area of a pizza. Later on we'll learn how to obtain the value from the user, so for now, let's assume that we know that the radius is 4.
- Radius: 4
- Area of circle: πr²
- Square of r:
4*4
or4**2
We know that the area of a circle is πr². We know two ways of squaring 4: we can either multiply 4 and 4 using a single star or we can take 4 to the power 2 using exponentiation. But what about π? In Python, π is part of the math module.
Using a module
Let's take a look at how to use a module. The keyword “import” is used to request that a module be used in a program. If you are using a module, this should be the first line of your program. To use a function that is in the module, use dot notation to join the name of the module with the name of the function. math.sqrt
is the square root function and math.fmod
is the modulo function. The following code
import math
print(math.sqrt(16))
print(math.fmod(14, 12))
gives the output
4.0 2.0
It is also possible to list the contents in the module using dir
; as a short form of “directory”. The following code
import math
print(dir(math))
gives the output
['__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'copysign', 'cos', 'cosh', 'degrees', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'hypot', 'isfinite', 'isinf', 'isnan', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'log2', 'modf', 'pi', 'pow', 'radians', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'trunc']
The first few listings are for types of information stored in the module. Each of these starts and ends with two underscores. You will see the underscores again as a special marker. Also in the list are names of functions, with familiar names like cosine, sine, and tangent in them, and also the constant π. To use the constant, we again use the name of the module followed by a period followed by the name of the constant. The following code
import math
print(math.pi)
gives the output
3.141592653589793
We can see that the number of digits following the decimal point is quite small, since this is an approximation of π as a floating point number. If you are using a few functions or constants again and again, you might choose instead to import a few individually instead of the whole module. In that case, you can use the name of the function or constant without the name of the module. The following code
from math import sqrt
print(sqrt(16))
gives the output
4.0
But remember you cannot use other functions that you haven't imported. The following code
from math import sqrt
print(sqrt(16))
print(fmod(14, 12))
gives the output
4.0 Traceback (most recent call last): File "<string>", line 3, in <module> NameError: name 'fmod' is not defined
As you can see from the error message, this name is not recognized. Fixing the name to use the name of the module doesn't help, since we haven't loaded the module. The following code
from math import sqrt
print(sqrt(16))
print(math.fmod(14, 12))
gives the output
4.0 TTraceback (most recent call last): File "<string>", line 3, in <module> NameError: name 'math' is not defined
Hence, as the error message indicates, the name “math” is not recognized.
Using a module: what we learned
To summarize, we import a module by typing import followed by the name of the module:
import math
If a module is being used, this should appear as the very first line of a program.
Once we've done that, we can use functions or constants from a module by putting the name of the module in front of the function or constant:
print(math.sqrt(5))
print(math.pi)
We can use dir
to get a listing of all the functions in the module:
print(dir(math))
If we only need to use a few of the functions or constants frequently, it might make more sense to import them individually so that we don't have to type the name of the module each time:
from math import sqrt
print(sqrt(5))
Caution
The module name is not dropped when the module is imported.
Just make sure not to omit the module name in the first case, when the module is imported.
Some contents of the math module
The following tables summaries some of the constants in the math module as well as the constant π.
Function | Pseudocode | Python |
---|---|---|
square root | squareroot(16) | math.sqrt(16) |
modulo | modulo(14, 12) | math.fmod(14, 12) |
Constant | Python |
---|---|
π | math.pi |
I've listed them the way they would be used if the module is imported. If they are imported individually, you would drop the math and the period from the front of each. Notice that the names are not quite the same in pseudocode and Python. I'll be showing tables like this in the future as well.
More common math functions
There are actually quite a few math functions that do not require the use of the math module. Here are a few we discussed.
Function | Python |
---|---|
exponentiation | pow(2, 4) |
absolute value | abs(-3) |
I haven't listed pseudocode since I didn't express them in pseudocode. In future you may see tables where some entries have pseudocode equivalents and some don't. Don't worry about trying to learn all the math functions in Python. Trying to learn a programming language by first mastering all the built-in functions is like trying to learn a human language by memorizing words in the dictionary.
What built-in functions are there?
"From scratch" promise
You will be shown a function before being asked to use it.
The focus of the course is the equivalent of learning how to start forming sentences and paragraphs. When you understand the structure of the language, you can start picking up words on your own. This course really is about learning from scratch: it is self-contained, providing you with what you need when you need it. But if you do want to start learning on your own, here are the tools:
The function dir
(short for directory), gives a list of the names of all the functions that apply to a particular data item, say the number 97:
print(dir(97))
To find out the names of all the built-in functions for all different types of data, the input to dir
is two underscores, builtins, and then two underscores:
print(dir(__builtins__))
Yes, you're seeing the two underscores again, as promised. But the name of a function alone doesn't do much good. Associated with each function is some explanatory text, called a "docstring" in python, where "doc" is short for documentation:
print(abs.__doc__)
A docstring is information stored with a function. You can print a docstring to see the information. Notice that dot notation is used here and again we see two underscores on each side of doc. The help page, which you can access from the menu on each page of the course, gives links to other resources where you can learn more about Python.
Example: determining the area of a circle of radius 4
It turns out that there are exponentiation functions both built in and as part of the math module. Each of these expressions multiplies π and the square of 4. Here are four different ways of squaring 4: multiplying 4 and 4, using the double star to calculate an exponent, using the function pow, and using the function pow in the math module. The following code
import math
print(math.pi * 4 * 4)
print(math.pi * 4 ** 2)
print(math.pi * pow(4, 2))
print(math.pi * math.pow(4, 2))
gives the output
50.26548245743669 50.26548245743669 50.26548245743669 50.26548245743669
Does it matter which one we use? The following code
import math
print(pow(4, 2))
print(math.pow(4, 2))
gives the output
16 16.0
This example shows that there is a difference between these two pow
functions. Even with integer inputs, the second one returns a floating point number.
In our pizza example, the outputs are the same in all cases. The square of four can be determined in any of these ways: 4*4
, 4**2
, pow(4, 2)
, and math.pow(4, 2)
. The last one always produces a floating point number.
But in general, it is crucial to remember which types are consumed and produced by each function.
Caution
Make sure to know the types of data consumed and produced by a function.
Using the wrong type of data sometimes can result in an error message. Sometimes it just results in something surprising. So make sure you know the types of inputs and outputs required for each function you use or create. I'll let you know what types of inputs and outputs are used for each function I teach you. The help page gives more information on finding information about functions.