Storing and using information (Part 1)
What is a variable or a constant?
In our examples using built-in functions, often we had to type the same expression again and again. Wouldn't it have been easier to store the value in memory, as even a calculator can do?
A variable has two parts. It is both a name used to refer to some data and the address where the data is stored.
Definition
A variable is
- the name used to refer to some data and
- the address (location) where the data is stored.
Notice that this is not the same as a variable in mathematics. A constant also has two parts. It is both a name used to refer to some data and the address where the data is stored.
Definition
A constant is
- the name used to refer to some data and
- the address (location) where the data is stored.
These definitions sound remarkably similar. The difference is in how they are used. As the name suggests, for a variable, the address associated with the name can vary or change. This makes sense when the value is one that is likely to change. As an example, for a variable like the cost of living, the address can change. In contrast, for a constant the address stays the same. For a constant like π, the address does not change. We do not want to redefine π.
What is an address?
But what is an address? An address is a location in the computer's memory.
Definition
An address is a location in the storage space (memory) of a computer.
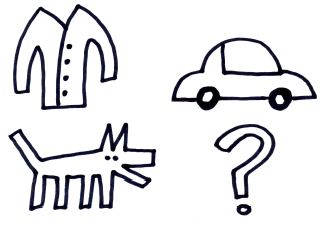
Image: Ivor Traber
The computer is able to store diverse kinds of data. You can think of it as a combination coat check, valet parking, and kennel, that is also ready to find storage for previously-unknown types of objects.
Using memory
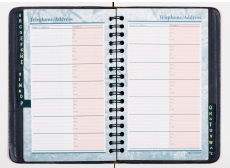
Image: circuler/iStock/Thinkstock
To use memory, we don't need to know details of how memory is organized or to what locations addresses correspond. All we need to do is specify a name for the storage space and possibly the type of data (such as a dog or a coat). The computer keeps something like an address book: it stores names with addresses.
The storing of data can be described in two steps. The first step is declaration, which is simply specifying the name of the variable (and, depending on the language, the type of data to be stored). The second step is providing the data to be stored. This is called initialization. The term assignment is more general, referring to any time a variable is given a value, whether or not it is the first time.
Definition
- Declaration: give the name (and possibly the type of data).
- Initialization: give the initial value.
- Assignment: give a value.
Some languages allow declaration and initialization to take place at the same time (in one step), and in many languages, the syntax for initialization is the same as the syntax for any other kind of assignment.
Memory use example
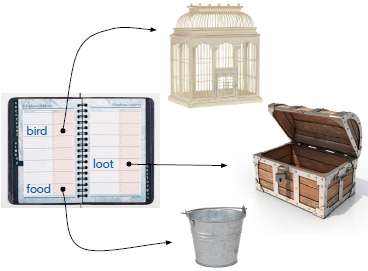
Fig. (a) Allocating space for the variables bird,
loot, and food
Suppose we wished to ask for space for a chicken using the name bird. We put the name bird in the address book and the address of the space. Here we use an arrow to indicate that an address is being stored, since we can think of an address as a way of "pointing" to a location. Now we ask for space for some money using the name loot. As a third declaration, we ask for space to store some snacks using the name food.
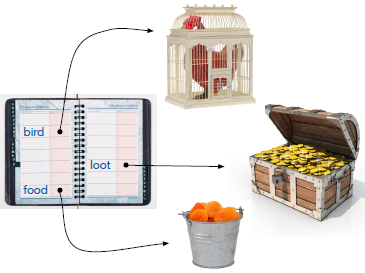
Fig. (b) Initializing the variables bird, loot, and
food by providing the values chicken, money,
and apricots, respectively.
We can now initialize the variables by providing values to store. We don't need to know the address, just the name. The computer can then look up the name in the address book and figure out where to put the item. Here we provide a chicken and ask to store it as bird. Now we provide some money and ask to store it as loot. Now we provide some apricots and ask to store them as food.
Suppose we want to assign the name food to some cranberries. We do not place the cranberries in the space allocated for the apricots.
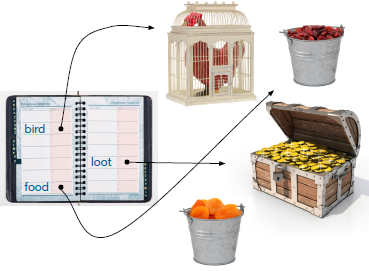
Fig. (c) Assigning the name food to cranberries.
Images (clockwise from top): cage/chicken -- rgbdigital/iStock/Thinkstock;
cranberries -- sarahdoow/iStock/Thinkstock; chest/coins -- koya79/iStock/Thinkstock;
apricots/cranberries/bucket -- sarahdoow/iStock/Thinkstock;
address book -- circuler/iStock/Thinkstock
Instead, we allocate new space for the cranberries, put the cranberries there, and change the address stored in the address book.
You may be worried about the apricots and you should be: there is no way we can get to them. In computers, the space used by such items is reclaimed by a process called garbage collection. Alas, the data itself is lost.
Declaration and initialization in different languages
Different languages use different syntax for declaration and initialization. Don't worry about the details of these examples: we will not be learning all the languages shown here.
In some, the type of the variable needs to be specified, as in this example where "int" denotes "integer":
int i;
i = 1;
In others, it suffices to give the name without declaring the type:
var i = 1;
Sometimes no explicit declaration is required:
i = 1
In general, there is a tradeoff. The more restrictions, the less flexiblity, but the easier it is to catch errors.
Types of languages
- More restrictive is less flexible but easier to catch errors.
- Less restrictive is more flexible but harder to catch errors.
Assignment in different languages
You may notice from these examples that in many programming languages, the same syntax is used for both initialization and assignment. A common way of showing assignment is using an equal sign or a slight variant. This can lead to confusion: it means a different symbol needs to be used for equality, and it makes it seem as if the left side and right side are symmetric, which they are not.
Consider the way the value 1
is assigned to the variable i
in some languages. In Python, Ada, AppleScript, XQuery, and F#, this is accomplished using i = 1
, i := 1
, set i to 1
, let i := 1
, and i <- 1
, respectively.
In our pseudocode, we will use a left arrow and omit declaration: i ← 1
Variables and built-in functions
To use variables in built-in functions, we use the name of the variable to represent the value that is stored. For example, suppose we assign the values 4 and 2 to variables x and y, respectively. In pseudocode this is written as
x ← 4
y ← 2
To determine the value of the expression x plus y, the computer looks up x and y in the address book, copies the values and adds them to get 6. Hence, the pseudocode
print(x + y) outputs 6.
We can use any of the syntax for function calls. For example, the pseudocode
print(power(x, y)) outputs 16.
In the following pseudocode, the computer tries to look up z in the address book but does not find it. That results in an error:
print(x + 2 - y + z) results in Error
In the following pseudocode, variable names are mixed with numbers:
print(power(x, 2) - x) gives 12
Notice that x is used twice.