Storing and using information (Part 2)
Pros and cons
Before exploring memory use further, it is worth taking a moment to discuss the pros and cons of this description.
Caution
- The view of the address book is a useful one, but don't expect to now have a detailed understanding of how computer hardware works - we've kept it simple by leaving out many details.
- Moreover, although this is a good description for our purposes, you shouldn't expect that all programming languages will work in exactly this way.
Pseudocode and memory example
With that in mind, let's see how we can use pictures to show what is going on in memory. In the left column is pseudocode, in the right column, a picture of memory. The dotted vertical line in the picture is used to distinguish between the names of the variables (like the entries in the address book) and the values stored in memory. Consider the sequence of pseudocode given below executed in the order listed.
-
Here the variable x stores the value 10.
-
If we then assign x to 20, we place 20 in a new location in memory and update x's arrow to point to the new location. This is like the example with the cranberries: we need to allocate new space for a new value.
-
When a variable name appears alone to the right of an arrow, it represents the address associated with that variable name. Thus, assigning x to y means storing the address of x as the address of y.
-
If we now reassign x to a new value 30, again we allocate memory for 30 and update the address associated with x. Notice that the address associated with y does not change. This shouldn't be a surprise, since the address of y will change only when y appears to the left of an arrow in an assignment. When a variable name appears in an expression on the right side of the arrow, it represents the value stored in the location associated with that variable.
-
Here we are being asked to add 20 to 5 and then to store the result in a new address associated with z.
-
30 ← y:
What does this mean? Nothing at all. An assignment always has to have a variable on the left side. If we had wanted to assign the value 30 to y, we should have written y ← 30 instead.
-
z ← w:
What does this mean? It is asking the address of z to be changed to the address of w. But when the computer looks in its address book (that is, the left side of the dotted line in the picture), it finds no entry for w. This will result in an error.
Incrementing a variable
It is common for a program to increment a variable. Here we have a variable x with the value 5.

x ← 5
We now make the assignment
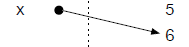
x ← x + 1
How do we interpret this assignment statement? Remember that a variable name in an expression on the right side of the arrow means the value stored in the address associated with that variable name. So the right side has the value 5 + 1 or 6. The presence of x on the left side of the arrow means that it is the variable name whose address is being updated. In particular, memory is allocated for the value 6 and the arrow from x is updated to point to that new address.
Summary of the use of variables in assignments
Here's a way to remember what variable names mean on different sides of the arrow. The name on the left of the arrow is the name of the variable that is getting a new address. The right side of the assignment statement gives an address to be reused or a value to be stored. Any variable name used here must already appear in the address book. On its own, it gives the address to be reused. As part of an expression, it supplies a value to be used.
The variable on the left side gets a new address.
It means “change the address of this variable.”
A variable name on the right side must appear in the address book.
- Alone, it means “use the address.”
- In an expression, it means “use the value found at the address.”
Actually, this last statement is not always true, as we will see in the future. But it will be true up until you are told about the other cases, so for now, pretend you never had this glimpse of the future.
Glimpse of the future
In an expression, a variable name on the right side may also mean “use the address.”
Keeping track of variables
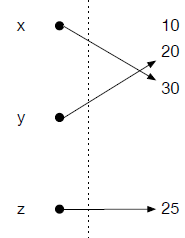
In writing programs, make use of pictures to keep track of the changes to variables so you always know what values are being stored.
- Use drawings to help you visualize what is happening.
- Update your drawing at each step.
Naming variables
The technical term for the name of a variable is an identifier.
Definition
An identifier is a name that you choose for a variable or other creation.
How do you choose a good name? Humans are pretty demanding creatures, expecting a consistent style in the choice of names and for variable names to convey meaning about the value stored and its type. So use use a consistent style and indicate the kind of data it is and how it is to be used.
Throughout this course, we'll talk about conventions. Conventions are not rules for the computer, hence not required for correctness, but rather suggestions on what will make humans happy. The computer just follows orders without worrying about the meaning, so it is less demanding. It just wants to be sure that there is no ambiguity to confuse it, so that words with special meaning in the computer language, called reserved keywords, are to be avoided.
Good and bad choices
Let's make some attempts to come up with a good name for a variable.
Attempt 1: x
Typically, x is not a very descriptive choice. It does not convey the meaning of the variable.
Attempt 2: print
print is usually a reserved keyword. We avoid such words.
Attempt 3: TheAmountOfMoneyIGetPaidEachMonthThatIWorkAtThisJob
This is an example which conveys a lot of information, but at the cost of too much reading and writing due to its excessive length. So this is not a good choice.
Attempt 4: Salary
Salary is a good choice.
Let's put our new knowledge to work by revisiting the room painting example.
Example: painting rooms, revisited
The problem was more understandable when we broke up the calculation into smaller steps, so we'll make use of variables to store values from these steps. The calculation in the painting example is given below.
The area of all rooms = 20 × The area of one room
The area of one room = 2 × ( The area of big wall + The area of small wall )
The area of the big wall = 8 × 10
The area of the small wall = 6 × 10
We can use the short but descriptive names all, one, big, and small. But translating these into pseudocode gets us into trouble.
all ← 20 * one
one ← 2 * (big + small)
big ← 8 * 10
small ← 6 * 10
Do you see why? In the very first assignment statement, we have the name one on the right side. But one isn't in the address book yet. We can fix this problem by choosing an order so that no variable ever appears on the right side until it has been assigned a value:
big ← 20 * 8
small ← 6 * 10
one ← 2 * (big + small)
all ← 20 * one
Notice that big and small could have been initialized in either order.