Storing and using information in Python (Part 1)
The double-edged sword
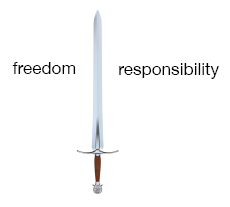
The syntax that Python has for dealing with variables is really simple, which is both good and bad. We can view the situation as a double-edged sword: Python allows a lot of freedom, which is a good thing, but with that freedom comes responsibility, which, although not a “bad” thing, in this context means more work.
Pros and cons of Python
When weighing the pros and cons of how Python is designed, flexibility belongs in both lists.

iStock/Thinkstock
Flexible:
Shortcuts to express meaning

iStock/Thinkstock
Flexible:
Multiple meanings
Best of both worlds: habits that make errors easy to catch.
There may be less to write, but more danger of not expressing the intended meaning. Operator overloading is one example of this problem. In contrast, when everything is pinned down and the meaning is clear, you can easily detect an error. In our programming, we will take advantage of the flexiblity without letting it cause us problems, in particular with good habits that make it harder for errors to go undetected.
Python syntax
Language feature | Pseudocode | Python |
---|---|---|
Declaration and initialization | count ← 5 | count = 5 |
Assignment | count ← 8 | count = 8 |
In Python, declaration and initialization are easy: give the name of the variable, write an equals sign, and then write the value. Exactly the same syntax is used to assign a new value to the variable later in the program.
Caution
The sign =
does not mean equals in Python.
Pay extra attention when using the equals sign. It does not represent equality.
Caution
The left and right sides of =
have different meanings.
No explicit constants
Another way in which Python syntax is simple is that variables and constants are created and used in the same way.

iStock/Thinkstock
You don't have to write anything
extra to create a constant.

iStock/Thinkstock
You might change the
value of a constant.
This means that there is less syntax to memorize, but there is also nothing forcing you not to change a constant. How do we solve the problem?
Python convention
Use all capitals for names of constants.
We capitalize names of constants to help us remember not to change them. For example, the number of days in a week should not change: DAYS_IN_WEEK = 7
.
Variable types are not specified
Unlike in the example that we asked for storage space for a bird that was shown as a cage or storage space for money that was shown as a chest, here we do not specify what type of data is stored.

iStock/Thinkstock
Change a variable's type.

iStock/Thinkstock
Using a function on the
wrong type is easy.
This gives us the freedom to change the type of data stored in a variable, or using different types in different circumstances, for example, sometimes an integer and sometimes a string. But this also gives us the freedom to use functions on the wrong type of data, when we make incorrect assumptions about the type.
Good habit
Use comments as reminders of the type.
We solve the problem by using comments to remind ourselves. To write text that the computer will ignore, use the sign #
. The computer will ignore anything that follows the #
sign to the end of that line. In this example, the comment is on a line by itself:
# age is an integer
age = int(input("Enter your age: "))
Declare and initialize simultaneously
There is no extra syntax to remember for initalization or declaration; in fact, the only syntax needed is that for assignment.

iStock/Thinkstock
Write both succinctly.

iStock/Thinkstock
Accidentally lose values.
This makes it easy to express, but also to accidentally loose a value by assigning a new value to a variable that you thought you were using for the first time, like in this example:
cat = 5
cat = 7
Good habit
Use comments and/or notes to keep track of meanings of variables.
Again, we can use comments to help with this problem. Another fine method for making work productive is planning and note-taking away from the computer during development.
Rules and conventions for identifiers
In choosing names for constants and variables, remember that you need to keep the computer happy and want to keep humans happy. You will see an error message if you break any of these rules.
Syntax
- Use letters, numbers, and underscores.
- The first character cannot be a number.
- No keywords.
Form your identifier mostly from letters with numbers and under scores as needed, though not starting with a number. Also, don't use a Python keyword. You can find more information about these on the help page.
Optional information
In newer versions of Python, some other characters are allowed.
Actually, there are a few more character that are allowed in newer versions of Python, but we won't be using them in this course.
Python convention
Variable names are in lower case with underscores used to separate words, as needed; constant names use upper case instead of lower case.
So as far as the computer is concerned, you could have an identifier made entirely of underscores, but for the sake of humans, you'll restrict yourself to lower case letters for variables, upper case letters for constants, and judicious use of underscores for readability.