Creating functions (Part 3)
Function calls and flow of control
What happens when we call a function?
Definition
The flow of control in a program is the order in which instructions are executed.
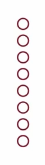
Fig. (1)
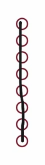
Fig. (2)
Figure (1) is a representation of a program. The program is represented using a column of eight identical red circles. Each red circle represents an instruction. If there are no function calls or other special instructions, the first instruction is executed first, the next one next, and so on from top to bottom. The completion of the execution of the instructions is represented by a line drawn from the top circle to the bottom circle, as shown in figure (2), in the order instructions are executed.
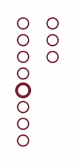
Fig. (3)
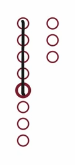
Fig. (4)
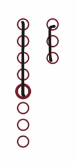
Fig. (5)
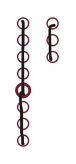
Fig. (6)
Figures (3)-(6) represents a program that calls a function during its execution. Each figure consists of two columns of red circles, the first column represeting the main program and the second column representing the function called by the program. The first column of red circles consists of four identical red circles, followed by a larger red circle and another set of three identical red circles. A larger red circle is used to represent a function call.
Here's how the program is executed: the instructions are executed until the function call is reached. In figure (4), this is reprsented by the line drawn from the top circle to the larger red circle. Then the instructions in the function body are executed. This is represented in figure (5), where a line is drawn from top circle to the bottom in the second column of red circles. Finally, the rest of the steps that appear after the function call are executed. This is shown in figure (6) by a line drawn from the larger red circle to the bottom red circle in the first column of red circles.
Function calls and address books
During the function definition, the function name is added to the address book:
Since a function definition assigns a name to a function, we add the name to our address book. That means that we cannot have a function and a variable with the same name. At least not in the address book we've discussed.
During a function call, a temporary address book is created:
When a function call occurs, a special temporary address book is created. We use this for the name of the parameter and the names of any variables defined in the function body, like big and small in our wall-painting example.
To look up a name,
- use the temporary address book, and
- if needed, use the permanent address book.
When a name is used in the function body, the computer first looks for the name in the temporary address book. If it isn't there, then it looks in the permanent address book. If this sounds a bit confusing, don't worry: we'll look carefully at an example in just a moment.
After a function call, the temporary address book disappears:
You may have been wondering why I called the address book temporary and now you know why: after the function call ends, it disappears. You may have wondered what would happen if the same name appeared in both books. Since during the function call the computer looks in the temporary address book first, the one in the permanent address book will not be found. Which sometimes is fine - you just need to be aware of it.
Caution
If the same name is used in both books, the one in the permanent address book will not be found.
Calling a user-defined function
Let's go through a little example, showing what happens in memory during each step.
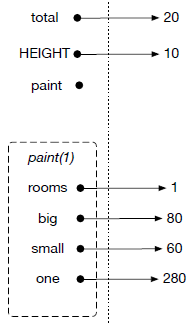
Fig. (7)
total ← 20 HEIGHT ← 10 define paint(rooms) big ← 8 * HEIGHT small ← 6 * HEIGHT one ← 2 * (big + small) return rooms * one single ← paint(1) many ← paint(total)
First, we assign the value 20 to the variable total, which gives us the name total in our permanent address book. Next, we create a constant HEIGHT with value 10. Our next step is to define the function, which results in the name of the function being placed in our permanent address book. Notice that defining the function is not the same as calling the function. We haven't yet asked to use the function paint. In our next step, we call paint on the input 1. Since rooms is the name of the parameter and since we are using the value 1 as the input, we put the name rooms in our temporary address book and give it the value 1. Since big is defined inside the function body, it too is placed in the temporary address book. To calculate the value, the computer looks up the name HEIGHT. Since it cannot find it in the temporary address book, it looks in the permanent address book to find the value 10, which it multiplies with 8 to obtain 80. The name and value of small are entered in the same way, though this time 10 is multiplied by 6 to obtain 60. To determine the value of one, the computer needs to look up the values associated with the names big and small. It looks in the temporary address book first and finds them there. These series of events are illustrated in figure (7).
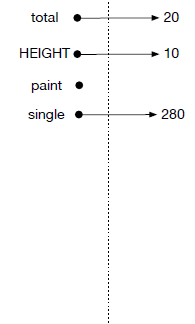
Fig. (8)
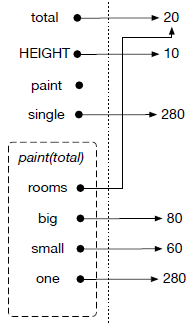
Fig. (9)
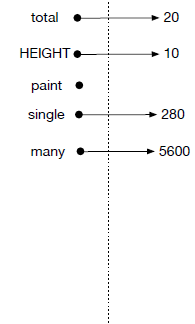
Fig. (10)
Now, rooms and one are multiplied, namely the values 1 and 280, and the value is given to the variable single. Since the function call is now over, the temporary address book has disappeared. These changes in memory are shown in figure (8).
We now have another function call, this time using the variable total as the input to paint. Again, we create a temporary address book. This time, the parameter name rooms has the same value as total. We calculate big as before, and also small, and finally one. These are shown in figure (9).
This is then multiplied by the value for rooms, namely 20, to obain the value 5600. Finally, this value is assigned to the new variable many. This is shown in figure (10).