Branching (Part 2)
Example: grading eggs
We've considered how to handle situations where there are two possible cases. But what if we have more than two cases?
Canadian eggs are categorized into six different sizes based on mass in grams, where we round to the nearest integer value:
Size | Mass in grams |
---|---|
Jumbo | 70 and over |
Extra Large | 63-69 |
Large | 56-62 |
Medium | 49-55 |
Small | 42-48 |
Peewee | 41 and under |
Dividing up all the possibilities
How might we write a program that prints an egg's size given its weight? Since branching splits all possibilities into two cases, we will need to branch more than once.
In the pictures below, the circle represents all possibilities. Branching splits the possibilities into two cases, those for which the condition is true (left half-circle), and those for which it is false (right half-circle). This is shown in figure (1). Although the two halves of the circle are of equal size in the picture, we are not restricted to the possibilities being split evenly. Here we can think of the left half circle corresponding to Jumbo eggs and the right half circle corresponding to everything else. As illustrated in figure (2), by using another condition, we can split the right group (right half circle) into two, such as the "Extra Large" eggs (left quarter circle) and everything else (right quarter circle). Again, the sizes of the pieces of the circle do not imply how many there are of each kind of egg.
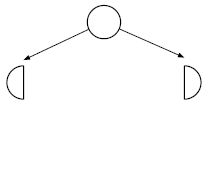
Fig. (1): Branching once
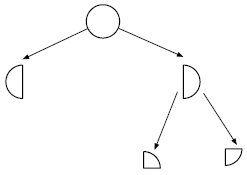
Fig (2): Branching twice
Using branching to grade eggs
Here is another visualization of the idea. Figure (3) below represents all chicken eggs in Canada.

Fig. (3)

Fig. (4)

Fig. (5)

Fig. (6)

Fig. (7)

Fig. (8)
Using the condition mass >= 70 separates out the Jumbo eggs from the rest. That is, they are the eggs for which the condition is true and for all the rest the condition is false. This is shown in figure (4). Since we know that all of the remaining eggs have weight less than or equal to 69, to distinguish between "Extra Large" and the rest, we just need to check that the mass is at least 63. So we use the condition mass >= 63. This is illustrated in figure (5). Here we are taking advantage of the fact that the first condition was false to give us extra information. We can use the same idea again to separate out the "Large" eggs: use the condition mass >= 56, as shown in figure (6). Any egg for which the first two conditions are false must have mass at most 62. So again we are using information from the earlier conditions to make a simpler condition than checking the value against the entire interval of masses. We use the same idea to separate the "Medium" eggs: we use the condition mass >= 49, as shown in figure (7). Now there remain only two cases to distinguish. So we choose a condition such that if the condition is true the egg is a "Small" egg and if the condition is false, the egg is a "Peewee" egg. This is the condition mass >= 42. This is illustrated in figure (8).
Creating pseudocode
Now that we know all the conditions, we can easily translate our ideas into pseudocode. Any egg for which the first condition is true is a "Jumbo" egg. We now introduce new syntax to allow us to ask a further question when the first condition is false: "else if". The words "Extra Large" will only be printed when the first condition is false, that is, the mass is at most 69, and the second condition is true, that is, the mass is at least 63. Similarly, "Large" is only printed when the first two conditions are false and the third condition is true. That means that the mass is at most 62 and at least 56. Again, remember that the lines under a condition are executed only when that condition is true and all previous conditions are false. The last two cases are printing "Small" when the first four conditions are false and the last condition is true, and printing "Peewee" when all five conditionsn are false. This now looks like our split between two cases, where we use "else" to separate them. The only difference is that the condition is introduced with "else if" instead of "if" since it is the fifth condition, not the first.
if mass >= 70 print("Jumbo") else if mass >= 63 print("Extra Large") else if mass >= 56 print("Large") else if mass >= 49 print("Medium") else if mass >= 42 print("Small") else print("Peewee")
More than two sets of instructions
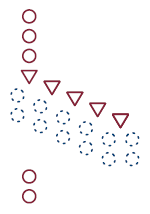
To use our red and blue circles visualization for this example, we observe that there are five triangles. For the first triangle, the choices are to go to the left, using the blue circles, or to go to the right. which leads to another triangle. So the leftmost blue circles correspond to "Jumbo", the next to "Extra Large", and so on. Pay special attention to the rightmost triangle. Both of the choices lead directly to blue circles and not another triangle. This corresponds to the condition "mass greater than or equal to 42" where the two options are printing "Small" and printing "Peewee".
Visualizing more than two sets of instructions
Let's use the diagram to show what the six possible courses of action are. There are six sets of blue steps. In each of the six cases, only one set of steps will be executed. In all cases, the upper and lower red steps will be executed. When the first condition is true, the first set of blue steps is executed and then the lower red, as shown in figure (9). When the first condition is false the second condition is checked, and if it is true, the second set of blue steps is executed, as shown in figure (10). When the first two conditions are false, the third condition is checked, and if it is true, the third set of blue steps is executed, as shown in figure (11). In figure (12), the first three conditions are false but the fourth is true. In figure (13), the first four conditions are false but the fifth is true. Finally, in the last case, as shown in figure (14), all five conditions are false.
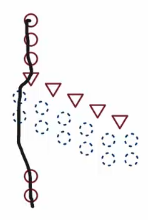
Fig. (9)
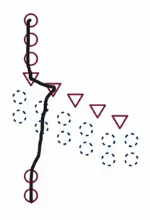
Fig. (10)
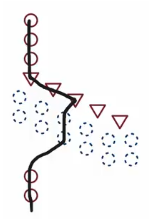
Fig. (11)
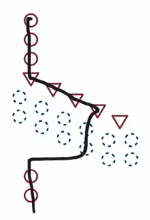
Fig. (12)
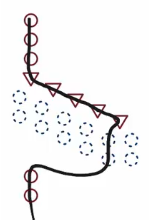
Fig. (13)
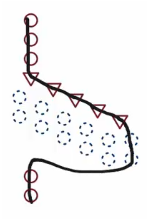
Fig. (14)